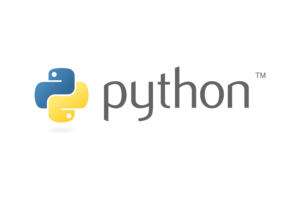
Introduction to Data Structures
Data Structures are used to store data in python when there is a need to organize data and access it efficiently. This is especially useful when you are working with large data sets. In the previous tutorial I briefly touched on the commonly used data structures within Python and how they are organized. Some common data structures in Python are:
Tuples
– Cannot be modified or changed after creation
– Good for storing historical data with indexing
List
– Good for storing values that may change
– Can be used to perform calculations using list comprehension
Dictionary
– Keys may not be duplicated
– Values / keys may be changed or removed
– Used when different data types need to organized under a common theme
Lets take about some use cases for each data structure:
Tuple – Used to store a list of test scores
List – Used to store a list of current ice cream flavors
Dictionary – Used to store a an individuals personal record (name, age, height, etc.)
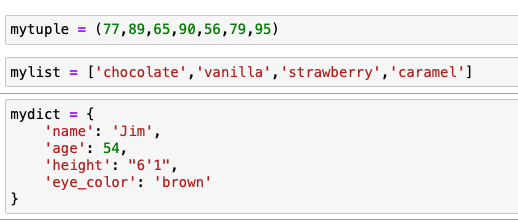
Now that we have set up the data structures, let dive into some functions that can be performed with the different types:
Tuple – Since a tuple is immutable, there isn’t much you can do with this data type except for selecting an element using an index, calculating the length of the tuple, or counting the number of times an element exists within a tuple
List – You can add an element to a list, remove an element, or select an element
Dictionary – Similar to a list, you can add, remove or select a key-value pair.
Starting with the variable “mytuple” created earlier, below you will see how those functions are performed in Python.

By using brackets, you can select an element from the tuple using an index number. Also one thing to note in Python is that an index starts at 0 instead of 1. In the case of mytuple, the index will start at 0 and end at 6. If you were to attempt to execute mytuple[7], it would return an index out of range error in Python. Additionally the len() function simply returns the length of the tuple. The count function returns the number of times “65” appears within the tuple.
Moving on to mylist, below you will see some functions that can be executed in Python.
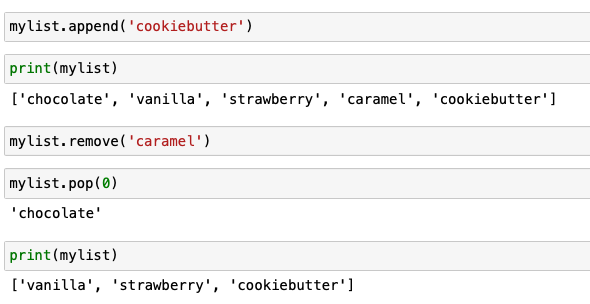
The append method add an element to the end of the list. The remove method deletes the specified element from the list. The pop method uses an index to the remove an element from a list. In the example above, ‘cookiebutter’ was added to the list, ‘caramel’ was deleted using the remove method and ‘chocolate’ was deleted using the pop method.
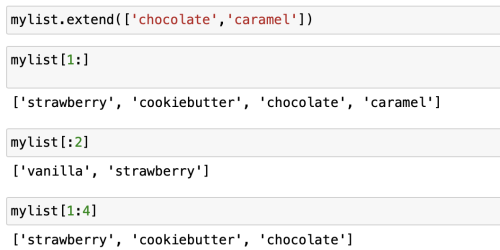
Let’s add back the flavors we deleted earlier. The extend method allows the ability to add multiple items to a list using the square brackets. Also you will notice there are multiple ways to select elements from a list. Adding a “:” after an index selects all items in a list after the specified index number. Adding a “:” before an index number selects all items starting from the beginning and ending at 1 minus the index number. In the second line above, using “:2” selected the first and second element from the list. The third line selected the second, third, and fourth item in a list. Now I know this may get confusing with Python starting an index with 0 but when selecting a range the last number in the index is subtracted by 1.
Finally, lets end with Dictionary methods.
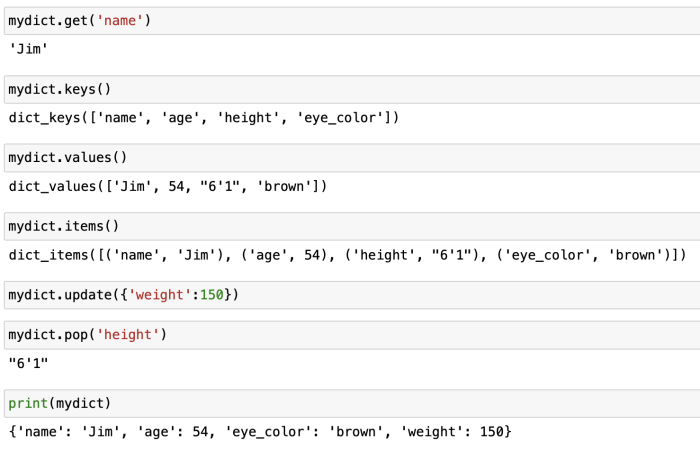
Dictionaries come in key value pairs. Each key is linked to a value and it usually describes the type of value it is. In the example above, the get method returns a value based on a key. The keys method returns all keys within a dictionary. The values method returns all values within a dictionary. The items method returns all key value pairs within a dictionary. The update method adds a new key value pair to the dictionary. The pop method deletes a key value pair based on a key name. In the example above, we added a key value pair for weight and removed the height.
Practice
Now that we have learned some data structure methods lets get into some practice:
Try to create a tuple of on your own using colors (blue, green, red, yellow, brown) and selecting a range of the first three colors.