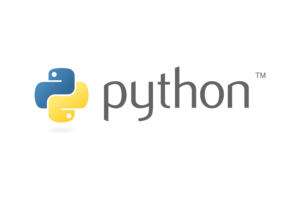
Introduction to Python
According to PYPL index, Python is still the most popular programming language. I like to think its because its so easy to use, has tons of support and is great for data analysis. Additionally, it’s very intuitive compared to other languages and can be used for many things including:
- Data Analytics
- Software Development
- Scripting
- Automating processes
- Games
Python is the second programming language I learned and one thing I noticed about Python as compared to other languages is the large number of 3rd party packages it offers and how easy it is to install them. Python packages are basically pieces of code developed by other software developers and can be used by anyone who wants to install them. An example of a popular python package is pandas which is used to create tables and manipulate data within the tables. Now you may wonder, why do I need pandas when I can just use Excel and formulas to manipulate data. While that may be true, pandas can process data much faster than Excel because Python is run in the background and can handle much larger amounts of data as compared to excel which last I checked had a limit of about 1 million rows. Before we dive into pandas, I wanted to provide a quick refresher on programming and how to install Python.
Installing Python
Installing python is a very straightforward process but it does take some time to install depending on how fast your computer may be. You can install Python either directly through Python.org or using Anaconda. I recommend installing Anaconda because it comes packaged with many third-party packages including pandas and other applications that can be used to execute Python code:
Once installed, depending on how Python was installed you should see a new application called “Python” or “Anaconda-Navigator” within your programs. If you are having trouble confirming if python was installed you can open up command prompt (Windows) or terminal (Mac) and type “Python” and press enter. If Python was successfully installed you should see python load within the window.
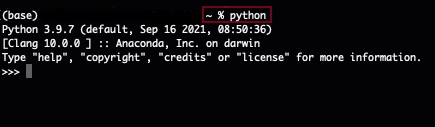
Jupyter Notebook
Jupyter Notebook is a web-based application that can be used to execute Python code. I personally love using it for data analytics when I am not sure how I want to manipulate data and would like to view the data prior to making changes. Jupyter Notebook differs from other applications because it allows you to run code line by line and retains the data in memory which saves time when you are dealing with large sets of data. In this tutorial we will be mainly using Jupyter Notebook but you are welcome to use any program you are comfortable with to follow along.
Quick tutorial: Jupyter Notebook can be launched either by searching for it in your program files or by launching Anaconda-Navigator and selecting Jupyter Notebook. Next you will click into the file directory you want to use to store your code and select New -> Python3 (ipykernel). This will create a blank python jupyter notebook file and differs from a normal python file which has a .py extension. To execute code you will type it into a cell and either click the run button at the top or shift+Enter. You can also insert, cut, copy or paste cells using the buttons at the top or shortcut keys (A – add, X – cut, C – copy, V – paste). Another cool feature I love about Jupyter Notebook is that it auto-saves your work.
IDE
An IDE stands for integrated development environment and is an application that is used to build software applications. This differs from Jupyter and CMD prompt because its provides additional tools for developers such as build automation tools, debugger, and additional add-ons. I personally use Visual Studio / Visual Studio Code as an IDE once I have tested my code in Jupyter Notebook and want to organize the code into methods and turn it into an executable. An IDE is great for organizing files especially if they are written in different programming languages.
Variables
Variables are essentially a method to store data within an object and are referenced later in the code. It’s really easy to assign variables in Python. See below for examples.
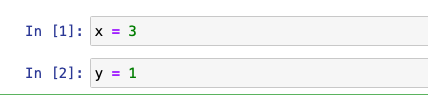
Once a variable is assigned it can be referenced later in the code, used in another function, or assigned to a new value.
Data Types
Python has several different data types but for the purposes of this tutorial we will discuss the following:
– String (Any set of characters representing text)
– Integer (Whole number)
– Float (Number with decimal)
– Boolean (True/False statement)
Python is a dynamically-typed language which means that the interpreter assigns a data type to a variable at run-time based on the variables value at the time. An example would be that if x = 3 then the data type would be an integer. If for some reason the value of x changed to “Hello!”, then the data type would change to string. Now this obviously has pros and cons but what this means is that you should be careful when creating variable names. Variables should be descriptive of the value they contain and should not be reused as this would increase the risk for an error and may cause confusion when reading the code. Addtionally, Python is also case sensitive which means that “myvariable” is not the same as “Myvariable”. When troubleshooting errors, sometimes the root cause is the incorrect case for a given variable or function.
Functions
Functions are the the basic building block of programming. They are used to execute a repeatable block of code and generally use variables to pass data and return a result. Functions can be created, imported from other packages, or can be executed by using Python’s built-in functions. Now you may wonder, why do we need functions when we can just write and execute code? Well, one of the key principles of programming is to don’t repeat yourself. This means that if you have to execute the same code over and over, you should try to be efficient by creating a function and call the function instead of retyping the same piece of code. This also helps to organize your code and reduces the need to re-invent the wheel when certain functions exists to perform a specific action. For the purposes of this tutorial, we will discuss how to call functions. A basic built-in function within python and one that is used very often is “print”. It’s a function that returns the output of a variable or any other value that is inserted within it. The print function is very useful when you are debugging code and attempting to identify the root cause of an error.
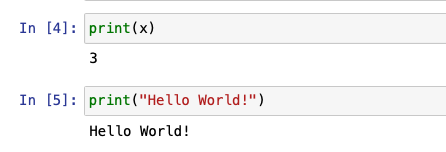
Data Structures
Data structures are used to organize data and can store large amount of data. Some common data structures in Python are:
– Tuples (Used to store a list of data that is ordered and unchangeable)
– List (Used to store a list of data that is ordered and changeable)
– Dictionary (Used to store a list of data in key: value pairs)
An example of how these data types are created can be found below.
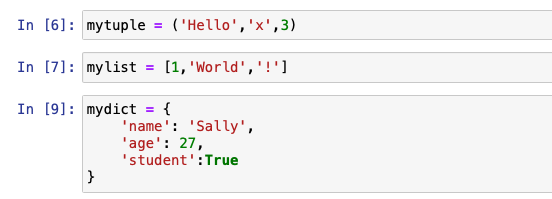
You can verify the data type by using the built-in type function in Python. See below:
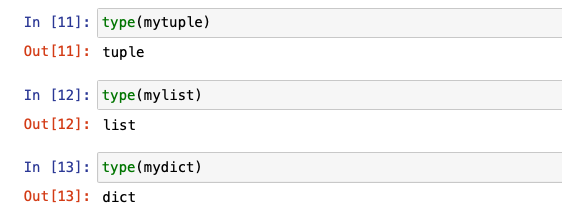
Operators
Operators are essential to programming and are used to perform calculations on variables and values. Some common python operators are:
+ (Used to add values together)
– (Used to subtract values)
* (Used to multiply values)
/ (Used to divide values)
= (Used to assign a value)
== (Used to compare two values to determine if they equal each other)
!= (Used to determine if two values do not equal each other)
> (Used to determine if a value is greater than another value)
< (Used to determine if a value is less than another value)
>= (Used to determine if a value is greater than or equal to another value)
<= (Used to determine if a value is less than or equal another value)
See below for examples of how these operators can be used:
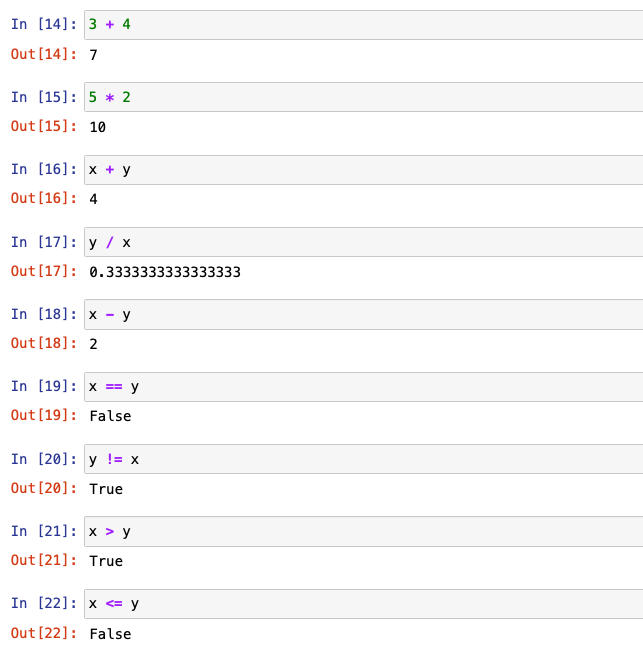
Comments
Comments are lines of code that are not executed at run time. They can be used to provide an explanation for the reader, provide instructions for running the code, or can be used to prevent certain code from running when testing code. There are two main methods of adding comments in Python:
# (Adding this character in front of any piece of code will create a comment for the remainder of the line)
”’ (Adding triple quotes can be used for multi-line comments)
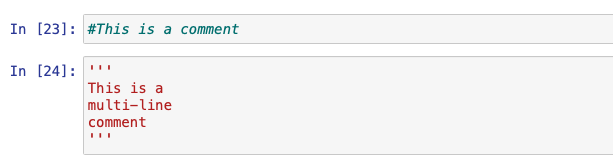
Practice
Now that we have covered the basics, let’s get some practice using variables and data structures. Try to do the following:
- Create a variable named z and assign it a value of 9
- Create a variable named y and assign it a value of 4
- Sum the two variables together and assign them to a variable named x
- Store all three variables in a list named mylist
You can click on the arrow below for the answer.
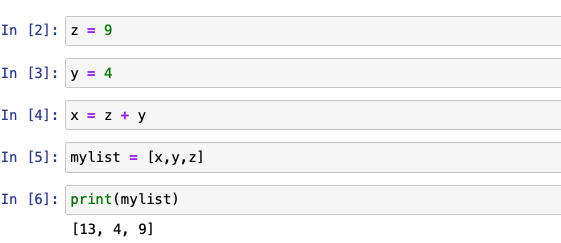
Summary
I hope this provided a brief overview of Python and the basic building blocks of programming. At the moment it may seem like there isn’t much you can do in Python but after learning the basics and getting familiar with Python packages, the possibilities are endless. I hope to continue with these tutorials and will dive into more practical applications in the future. If you would like to visit part 2 of this tutorial where I discuss data structures in more detail please click here.